how to make a calculator with graphical user interface(gui) in python
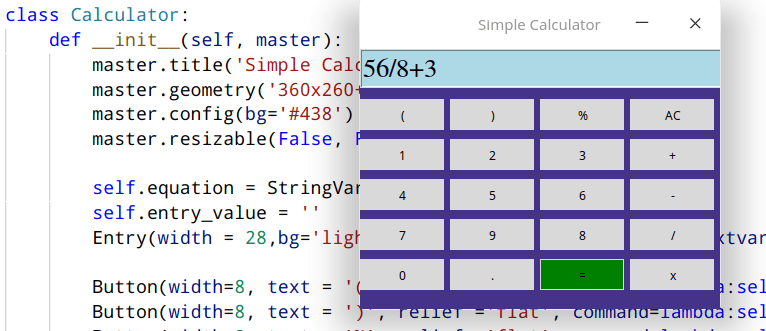
Hi guys,
In this tutorial, you're going to learn how to make your own fully functioning gui calculator in python using Tkinter in just a few minutes.
You don't need any additional library to completer to complete this tutorial other than Tkinter, which normally comes with python standard library utilities.
If your Linux you might need to install it
$ pip install python-tk
Now once everything is cleary installed let's start coding our calculator, at the end of the tutorial you gonna build something like this;

solving math using eval ( )
eval is a built-in- function used in python, it parses the expression argument and evaluates it as a python expression.
We are going to use the concept of eval to solve our mathematical expression.
Example of Usage
>>> while True:
... expression = input('Enter equation: ')
... result = eval(expression)
... print(result)
...
Enter equation: 2 + (9/9) *3
5.0
Enter equation: 12 /9 + (18 -2) % 5
2.333333333333333
With 4 lines of code you already made a command-line calculator in Python, now let's use the same concept to build a calculator with a graphical interface
There are three main parts of this GUI Calculator
- Screen to display expression (skeleton)
- Button holding values for expression
- Building a calculator logic
Making a skeleton for our calculator
from tkinter import Tk, Entry, Button, StringVar
class Calculator:
def __init__(self, master):
master.title('Simple Calculator')
master.geometry('360x260+0+0')
master.config(bg='#438')
master.resizable(False, False)
root = Tk()
calculator = Calculator(root)
root.mainloop()
Output :

1. Let's add screen to display the Expression
from tkinter import Tk, Entry, Button, StringVar
class Calculator:
def __init__(self, master):
master.title('Simple Calculator')
master.geometry('360x260+0+0')
master.config(bg='#438')
master.resizable(False, False)
self.equation = StringVar()
self.entry_value = ''
Entry(width = 28,bg='lightblue', font = ('Times', 16), textvariable = self.equation).place(x=0,y=0)
root = Tk()
calculator = Calculator(root)
root.mainloop()
Output :

Now we have have already done building our display screen , we have now have to add Button for forming a mathematical expression .
2. Adding Button for Forming Mathematical expression
These are button are being created in same way differing only value they hold and their geometrical locations, Button for forming mathematical expression includes
- 0 to 9 numbers
- Mathematical operator + , - , / , %
- Decimal point .
- brackets ( , )
We need to attach a command to each of these button so that when we click it , it shows on the display screen . I made a simple show ( ) just to do that.
from tkinter import Tk, Entry, Button, StringVar
class Calculator:
def __init__(self, master):
master.title('Simple Calculator')
master.geometry('360x260+0+0')
master.config(bg='#438')
master.resizable(False, False)
self.equation = StringVar()
self.entry_value = ''
Entry(width = 28,bg='lightblue', font = ('Times', 16), textvariable = self.equation).place(x=0,y=0)
Button(width=8, text = '(', relief ='flat', command=lambda:self.show('(')).place(x=0,y=50)
Button(width=8, text = ')', relief ='flat', command=lambda:self.show(')')).place(x=90, y=50)
Button(width=8, text = '%', relief ='flat', command=lambda:self.show('%')).place(x=180, y=50)
Button(width=8, text = '1', relief ='flat', command=lambda:self.show(1)).place(x=0,y=90)
Button(width=8, text = '2', relief ='flat', command=lambda:self.show(2)).place(x=90,y=90)
Button(width=8, text = '3', relief ='flat', command=lambda:self.show(3)).place(x=180,y=90)
Button(width=8, text = '4', relief ='flat', command=lambda:self.show(4)).place(x=0,y=130)
Button(width=8, text = '5', relief ='flat', command=lambda:self.show(5)).place(x=90,y=130)
Button(width=8, text = '6', relief ='flat', command=lambda:self.show(6)).place(x=180,y=130)
Button(width=8, text = '7', relief ='flat', command=lambda:self.show(7)).place(x=0,y=170)
Button(width=8, text = '8', relief ='flat', command=lambda:self.show(8)).place(x=180,y=170)
Button(width=8, text = '9', relief ='flat', command=lambda:self.show(9)).place(x=90,y=170)
Button(width=8, text = '0', relief ='flat', command=lambda:self.show(0)).place(x=0,y=210)
Button(width=8, text = '.', relief ='flat', command=lambda:self.show('.')).place(x=90,y=210)
Button(width=8, text = '+', relief ='flat', command=lambda:self.show('+')).place(x=270,y=90)
Button(width=8, text = '-', relief ='flat', command=lambda:self.show('-')).place(x=270,y=130)
Button(width=8, text = '/', relief ='flat', command=lambda:self.show('/')).place(x=270,y=170)
Button(width=8, text = 'x', relief ='flat', command=lambda:self.show('*')).place(x=270,y=210)
def show(self, value):
self.entry_value +=str(value)
self.equation.set(self.entry_value)
root = Tk()
calculator = Calculator(root)
root.mainloop()
Output :
The output is calculator with button and when you click any of those button it's value will appear on the display screen.

Now only two buttons are remaining for our calculator to be complete , the Reset button to clear the screen and the = sign button evaluate the expression and display the result to screen.
3. Adding Reset and equal Button to our calculator
from tkinter import Tk, Entry, Button, StringVar
class Calculator:
def __init__(self, master):
master.title('Simple Calculator')
master.geometry('360x260+0+0')
master.config(bg='#438')
master.resizable(False, False)
self.equation = StringVar()
self.entry_value = ''
Entry(width = 28,bg='lightblue', font = ('Times', 16), textvariable = self.equation).place(x=0,y=0)
Button(width=8, text = '(', relief ='flat', command=lambda:self.show('(')).place(x=0,y=50)
Button(width=8, text = ')', relief ='flat', command=lambda:self.show(')')).place(x=90, y=50)
Button(width=8, text = '%', relief ='flat', command=lambda:self.show('%')).place(x=180, y=50)
Button(width=8, text = '1', relief ='flat', command=lambda:self.show(1)).place(x=0,y=90)
Button(width=8, text = '2', relief ='flat', command=lambda:self.show(2)).place(x=90,y=90)
Button(width=8, text = '3', relief ='flat', command=lambda:self.show(3)).place(x=180,y=90)
Button(width=8, text = '4', relief ='flat', command=lambda:self.show(4)).place(x=0,y=130)
Button(width=8, text = '5', relief ='flat', command=lambda:self.show(5)).place(x=90,y=130)
Button(width=8, text = '6', relief ='flat', command=lambda:self.show(6)).place(x=180,y=130)
Button(width=8, text = '7', relief ='flat', command=lambda:self.show(7)).place(x=0,y=170)
Button(width=8, text = '8', relief ='flat', command=lambda:self.show(8)).place(x=180,y=170)
Button(width=8, text = '9', relief ='flat', command=lambda:self.show(9)).place(x=90,y=170)
Button(width=8, text = '0', relief ='flat', command=lambda:self.show(0)).place(x=0,y=210)
Button(width=8, text = '.', relief ='flat', command=lambda:self.show('.')).place(x=90,y=210)
Button(width=8, text = '+', relief ='flat', command=lambda:self.show('+')).place(x=270,y=90)
Button(width=8, text = '-', relief ='flat', command=lambda:self.show('-')).place(x=270,y=130)
Button(width=8, text = '/', relief ='flat', command=lambda:self.show('/')).place(x=270,y=170)
Button(width=8, text = 'x', relief ='flat', command=lambda:self.show('*')).place(x=270,y=210)
Button(width=8, text = '=', bg='green', relief ='flat', command=self.solve).place(x=180, y=210)
Button(width=8, text = 'AC', relief ='flat', command=self.clear).place(x=270,y=50)
def show(self, value):
self.entry_value +=str(value)
self.equation.set(self.entry_value)
def clear(self):
self.entry_value = ''
self.equation.set(self.entry_value)
def solve(self):
result = eval(self.entry_value)
self.equation.set(result)
root = Tk()
calculator = Calculator(root)
root.mainloop()
Hope you find this post interesting, don’t forget to subscribe to get more tutorials like this.
In case of any suggestion or comment, drop it in the comment box and I will reply to you immediately.
To get the full code for the above article check it on My Github